Circuit Playground Express projects

Circuit Playground Express equipment
- 10 x mini NeoPixels, each one can display any color
- 1 x Motion sensor (LIS3DH triple-axis accelerometer with tap detection, free-fall detection)
- 1 x Temperature sensor (thermistor)
- 1 x Light sensor (phototransistor). Can also act as a color sensor and pulse sensor.
- 1 x Sound sensor (MEMS microphone)
- 1 x Mini speaker with class D amplifier (7.5mm magnetic speaker/buzzer)
- 2 x Push buttons, labeled A and B
- 1 x Slide switch
- Infrared receiver and transmitter – can act as a proximity sensor
- 8 x alligator-clip friendly input/output pins
- Includes I2C, UART, 8 pins that can do analog inputs, multiple PWM output
- 7 pads can act as capacitive touch inputs and the 1 remaining is a true analog output
- Green “ON” LED
- Red “#13” LED for basic blinking
- Reset button
- ATSAMD21 ARM Cortex M0 Processor, running at 3.3V and 48MHz
- 2 MB of SPI Flash storage, used primarily with CircuitPython to store code and libraries
- MicroUSB port for programming and debugging
- USB port can act like serial port, keyboard, mouse, joystick or MIDI
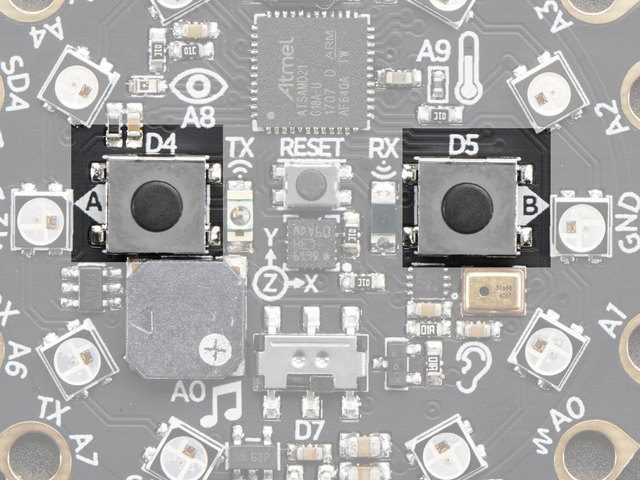
The Left button is connected to digital pin #4
The Right button is connected to digital pin #5
Both have 10KΩ pull-down resistors: button pressed means ‘high’ value.
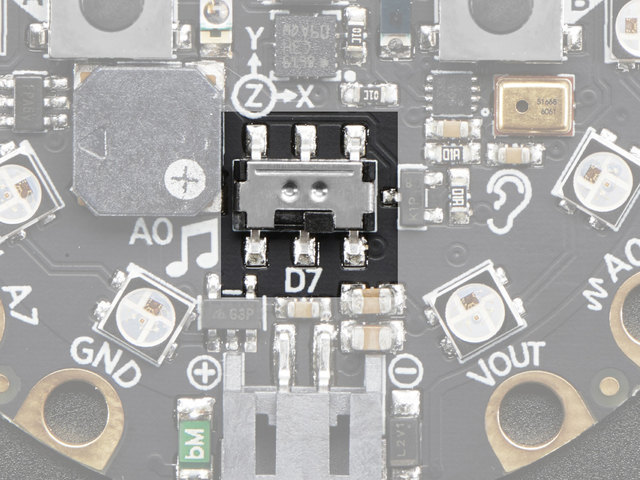
The slide switch is near the center, right above the JST Battery Jack.
This switch is connected to digital pin #7.
When switched to the left, the value read is ‘high’ (thus the + symbol) and when switched right, the value read is ‘low’ (- symbol).
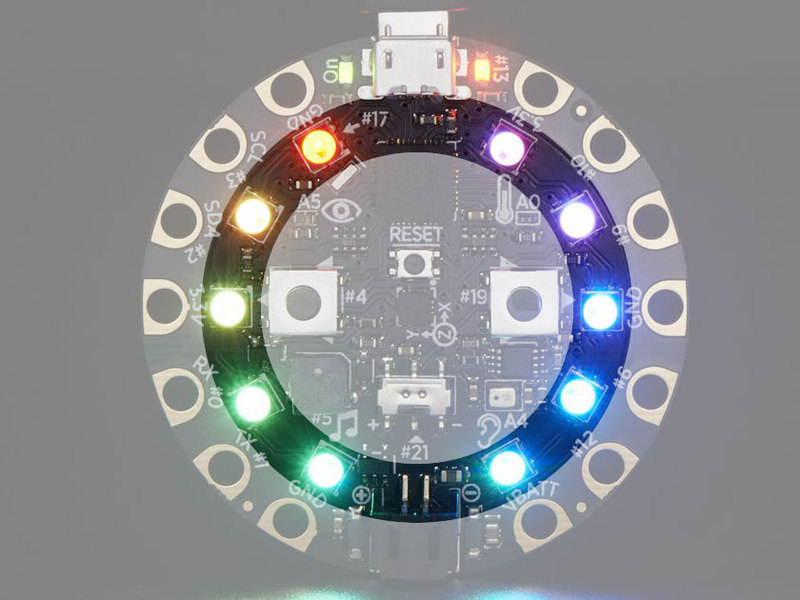
CPE has ten full color LEDs called NeoPixels that contain bright red+green+blue sub-LEDs.
These pixels are controlled in a chain by a single data pin, #17.
Unlike non-smart RGB LEDs, the programmer can set the color and a little chip inside the LED will handle the PWM.
Each NeoPixel is numbered, starting with #0 at the top left and going counter clockwise up to #9.
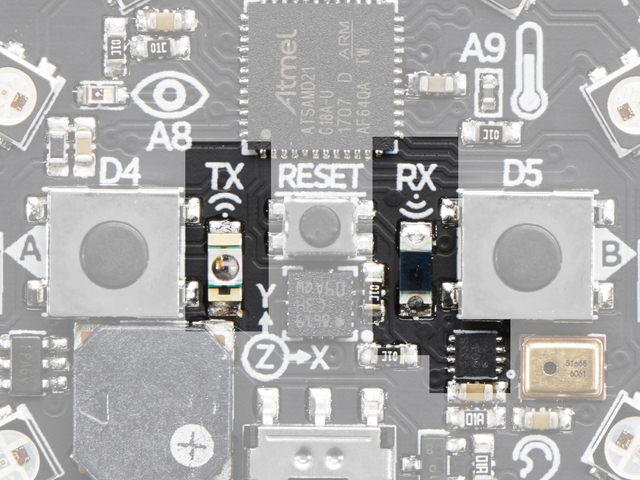
The IR transmitter and receiver on the Circuit Playground Express can be found near the center of the board.
The transmitter is labeled TX and is on the left side of the reset button, to the right of button A.
The receiver is labeled RX and is on the right side of the reset button, to the left of button B.
Programming CPE in Python
Infrared Receive and Transmit with Circuit Playground Express
Log your Circuit Playground Express data directly into a spreadsheet
NeoPixel library
To set the color for each pixel with the built in NeoPixel library:
CircuitPlayground.setPixelColor(n, red, green, blue)
where n is between 0 and 9 and indicates which LED you are setting. red/green/blue vary between 0 (off) and 255 (full on).
Circuit Playground Express and Raspberry Pi
Working with the Adafruit Circuit Playground Express using the Raspberry Pi 4 – Part 1
Working with the Adafruit Circuit Playground Express using the Raspberry Pi 4 – Part 2
Working with the Adafruit Circuit Playground Express using the Raspberry Pi 4 – Part 3
Working with the Adafruit Circuit Playground Express using the Raspberry Pi 4 – Part 4
CircuitPython Powered AT Hand-Raiser
CircuitPython Code
Python code on CPE checks with the CircuitPython Supervisor to see if there is any text to read from the USB port. If so, it calls input() to read the message (and then strips off any whitespace like newlines or spaces).
import supervisor # ... while True: # Check to see if there's input available (requires CP 4.0 Alpha) if supervisor.runtime.serial_bytes_available: # read in text (@mode, #RRGGBB, %brightness, standard color) # input() will block until a newline is sent inText = input().strip() # Sometimes Windows sends an extra (or missing) newline - ignore them if inText == "": continue